How to visualize MRI data?#
[1]:
from polpo.plot.mri import MriPlotter, MriSlicer, SlicesPlotter
from polpo.preprocessing.load import FigsharePregnancyDataLoader
from polpo.preprocessing.mri import MriImageLoader
from polpo.preprocessing.path import FileFinder, FileRule
Loading data#
We’ll create a pipeline that:
downloads data from figshare
finds nii file in folder
loads image as an array
[2]:
SESSION_ID = 1
[3]:
folder_name = f"ses-{str(SESSION_ID).zfill(2)}"
loader = FigsharePregnancyDataLoader(
data_dir="~/.herbrain/data/pregnancy/mri",
remote_path=f"mri/{folder_name}",
)
finder = FileFinder(
rules=[
FileRule(value="BrainNormalized", func="startswith"),
FileRule(value=".nii.gz", func="endswith"),
]
)
pipe = loader + finder + MriImageLoader()
[4]:
img_fdata = pipe()
Visualization#
MriSlicer
slices the MRI image. SlicesPlotter
takes care of plotting slices. By default, it plots side, front and top views.
[5]:
slices_plotter = SlicesPlotter()
slicer = MriSlicer()
[6]:
slices_plotter.plot(slicer.slice(img_fdata, (35, 70, 105)));
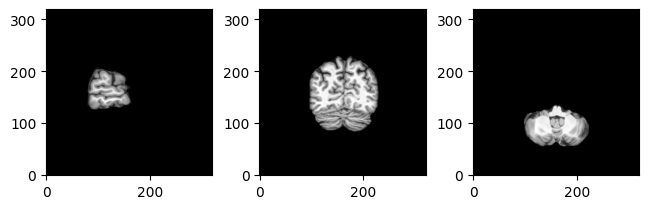
For convenience, there’s also MriPlotter
.
[7]:
mri_plotter = MriPlotter()
[8]:
mri_plotter.plot(img_fdata, [val // 2 for val in img_fdata.shape]);
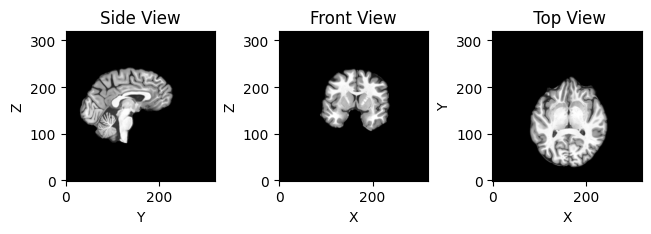